PydanticAI for Building Agentic AI-Based LLM Applications
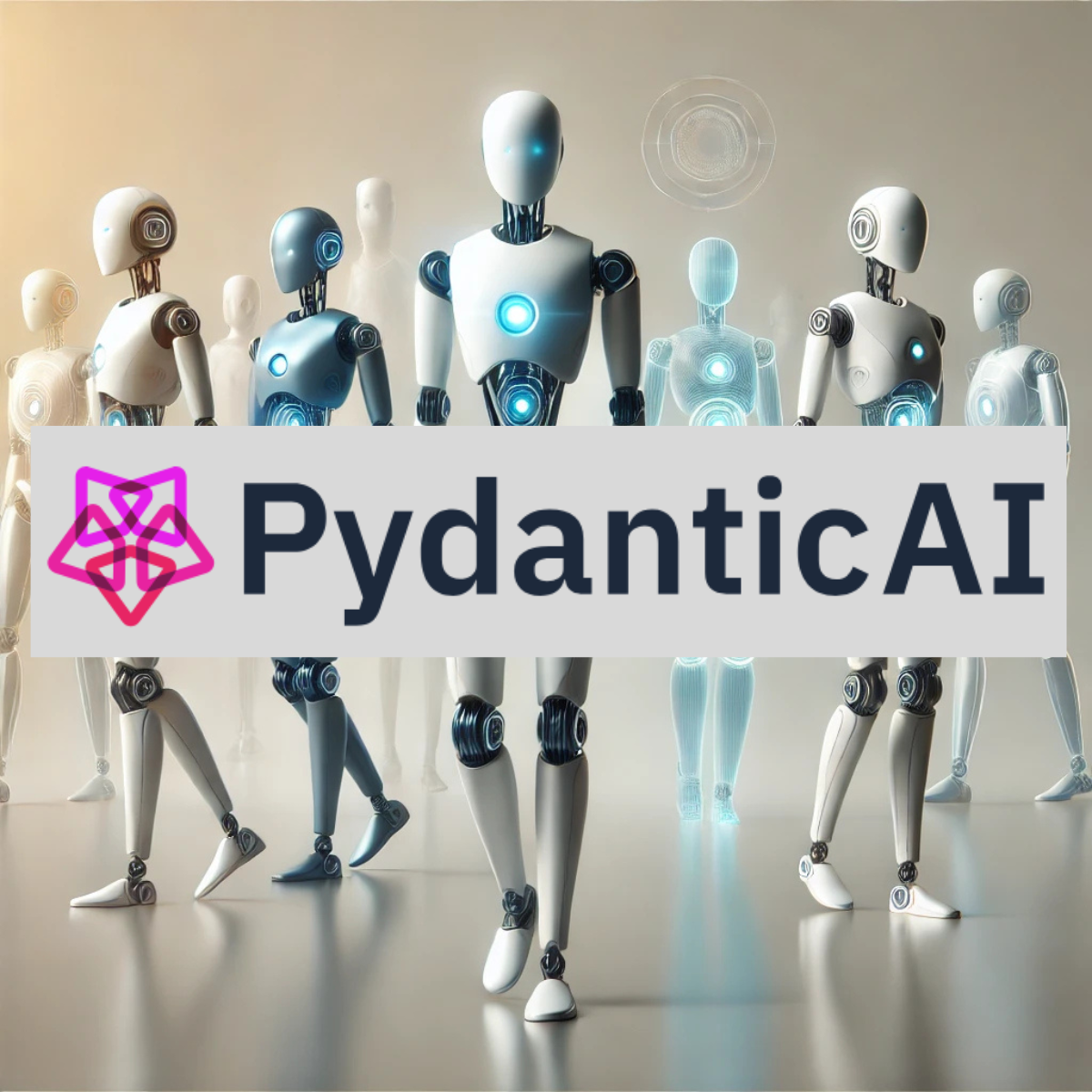
PydanticAI is a powerful framework designed to enhance the development of agentic AI-based applications using large language models (LLMs). Its model-agnostic nature allows developers to seamlessly switch between different LLMs, such as OpenAI and Gemini, without being restricted to a specific technology stack. In addition to this flexibility, the tight integration with the Pydantic library’s type-safe validation ensures that LLM outputs conform to expected data structures, significantly reducing errors and enhancing reliability in production environments.
The framework supports the entire application development lifecycle, offering features like dependency injection and integration with Logfire for effective debugging and monitoring. These capabilities make PydanticAI an essential tool for creating dynamic, type-safe AI applications that can interact with external systems and provide robust, real-time solutions in various domains, including customer support and interactive gaming.
Let’s take a look at how it works in more detail.
Primer on AI Agents
AI agents are autonomous software entities that leverage artificial intelligence to perform specific tasks or processes. They are designed to operate independently or collaboratively within a system to achieve defined objectives. Here's a breakdown of what AI agents typically encompass:
- Autonomy: AI agents can make decisions and act on their own based on input data, rules, or learned patterns.
- Specialization: Each agent is usually designed to handle a specific task or a narrow set of related tasks. For instance:
- Intent Detection Agent: Identifies the purpose behind a customer's query.
- Knowledge Base Search Agent: Retrieves relevant information from a database.
- Sentiment Analysis Agent: Analyzes emotional tone to adjust the response.
- Collaboration: In complex systems, agents can interact and share outputs, forming a pipeline or a DAG (like in the example diagram further ahead). This collaboration helps solve multifaceted problems efficiently.
- AI Techniques: They utilize machine learning models, natural language processing (NLP), or other AI technologies to execute their tasks.
Frameworks such as PydanticAI, langgraph, phidata, and OpenAI’s Swarm give developers a set of libraries to make complex application development easier.
In this article, we’ll focus on how PydanticAI handles agentic workflows.
Agentic Concepts in LLM Workflows with PydanticAI
PydanticAI's model-agnostic framework and type-safe validation streamline AI application development. That’s the value proposition, but does it live up to expectations?
This Python-based agent framework supports various large language models (LLMs) like OpenAI, Gemini, and Groq, with plans to include Anthropic. Its model-agnostic nature allows developers to switch between LLMs without being tied to a specific technology stack, enhancing flexibility and scalability. Covering all popular models is expected from any framework nowadays, so this is hardly a killer feature. But there’s more!
Type-Safe Validation for Robust Applications
PydanticAI's type-safe validation ensures that LLM outputs conform to expected data structures, reducing errors and improving reliability. This is crucial for production-grade applications where consistency is key. For instance:
- A bank's customer support agent can dynamically access customer data, offer tailored advice, and assess risk levels, ensuring data integrity through Pydantic's validation.
- E-commerce platforms can deploy agents to suggest products or process refunds, with every interaction validated against pre-defined schemas for seamless integration into backend systems.
- Healthcare applications can leverage agents to generate patient summaries or provide medication reminders while adhering strictly to structured data standards.
Features That Empower Developers
PydanticAI isn't just about compatibility; it’s a comprehensive development toolkit. The framework supports the entire application lifecycle, offering features such as:
- Dependency Injection: Simplifies application logic by managing dependencies transparently, reducing boilerplate code, and enhancing testability.
- Logfire Integration: Provides robust debugging and monitoring capabilities, essential for tracking performance and identifying issues in real-time.
- Native Python Support: Enables developers to write, extend, and maintain application logic using plain Python, avoiding the learning curve associated with domain-specific languages.
Scalability and Modularity
Beyond its core functionality, PydanticAI is designed for scalability. The modular architecture ensures that applications can grow alongside user needs:
- New agents can be seamlessly integrated into workflows without disrupting existing processes.
- Framework updates remain non-intrusive, (hopefully) ensuring long-term maintainability.
As you can see, PydanticAI proposes a fairly robust, developer-friendly foundation for building agent-driven LLM workflows, making it more than just another interoperability tool. Its focus on validation, lifecycle support, and scalability elevates its value proposition for AI-driven enterprises and definitely makes for a unique toolkit, compared to alternatives.
Real-World Applications with PydanticAI
As mentioned above, PydanticAI is spearheading the development of AI-driven applications by providing a robust framework for building scalable, type-safe systems.
The official documentation page provides various examples to showcase how the framework functions in real-world-like scenarios.
The first example is its use in customer support agents. For instance, a bank implemented a support agent using PydanticAI to access customer data dynamically, offer personalized advice, and assess risk levels. This agent, built with OpenAI's GPT-4o model, utilizes Pydantic's dependency injection to seamlessly integrate with live data sources, ensuring accurate and secure interactions.
Another application is in interactive games, where PydanticAI powers dynamic experiences. Developers can create games like quizzes or dice games, where responses are generated based on user input and predefined logic. This flexibility is achieved through PydanticAI's model-agnostic design, supporting various LLMs such as OpenAI and Gemini.
Of course, you have many options for writing such applications. So what makes PydanticAI stand out compared to other agentic frameworks?
- Type-Safe Validation: Ensures structured and predictable responses via tight Pydantic integration.
- Model-Agnostic Flexibility: Compatible with multiple LLMs.
- Dependency Injection: Simplifies integration with external data sources.
These capabilities make PydanticAI a powerful tool for developing reliable, production-grade AI applications.
Building AI Agents with PydanticAI
Even as a beta release, PydanticAI offers a robust framework for building AI agents with type-safe input/output handling and seamless integration with popular LLM providers.
To create an AI agent using PydanticAI, follow these steps:
1. Define the Agent: Use the Agent class to specify the LLM model, such as 'openai:gpt-4o' or 'gemini-1.5-flash'. Set the deps_type
and result_type
to ensure type safety. For example, an agent can be defined to check a roulette result using an integer dependency and returning a boolean result.
2. Register Tools: Use the @agent.tool
decorator to define functions that the LLM can call. These tools can access the agent's context via RunContext. For instance, a roulette_wheel
function can determine if a number is a winner based on external dependencies.
3. Run the Agent: Execute the agent using run_sync()
for synchronous tasks or run() for asynchronous operations. This flexibility allows for handling both simple queries and complex workflows.
Complete Example: A bank support agent can provide customer advice and assess risk levels by integrating tools and structured outputs. The agent dynamically accesses customer data and returns structured responses, ensuring reliable and actionable insights.
Here's a complete example agentic application. This example creates a simple agent that takes a city name as input and returns structured weather information, including temperature, conditions, and advice based on the weather.
from dataclasses import dataclass
from pydantic import BaseModel, Field
from pydantic_ai import Agent, RunContext
@dataclass
class WeatherDependencies:
"""Dependencies needed for the weather agent."""
weather_api_key: str # Replace with your actual API key
class WeatherResult(BaseModel):
"""Schema for the weather result."""
city: str = Field(description="Name of the city")
temperature: float = Field(description="Temperature in Celsius")
condition: str = Field(description="Weather condition (e.g., sunny, rainy)")
advice: str = Field(description="Advice based on the weather")
# Define the agent
weather_agent = Agent(
'openai:gpt-4o',
deps_type=WeatherDependencies,
result_type=WeatherResult,
system_prompt=(
"You are a weather assistant. Provide accurate weather information and "
"offer practical advice for the user based on the weather."
),
)
# Tool to simulate getting weather data (replace with real API calls)
@weather_agent.tool
async def fetch_weather_data(ctx: RunContext[WeatherDependencies], city: str) -> dict:
"""Simulates fetching weather data for a city."""
# In a real-world application, use an API like OpenWeatherMap
weather_data = {
"city": city,
"temperature": 24.5,
"condition": "sunny",
"advice": "It's sunny, wear sunglasses and stay hydrated!"
}
return weather_data
# Simulated run
deps = WeatherDependencies(weather_api_key="dummy_key")
result = weather_agent.run_sync('What is the weather like in Rio de Janeiro?', deps=deps)
print(result.data)
The important takeaways:
- Schema-Driven Results: The agent validates and structures output using Pydantic models, ensuring reliable and type-safe data.
- Extensible Design: Tools within the agent (like
fetch_weather_data
) enable integration with APIs or databases. - Simplicity: Pydantic-AI reduces boilerplate code, making it easy to build practical applications.
This example demonstrates how to utilize Pydantic-AI to create a functional, agentic app in just a few lines of code.
Comparing to Langchain
LangChain and Pydantic-AI are tools designed to assist developers in working with large language models (LLMs), but they approach this goal from different perspectives and serve distinct use cases. LangChain focuses on building complex LLM-powered workflows, while Pydantic-AI leverages Pydantic's data validation and schema tools to structure and validate LLM outputs.
Here, we'll compare the two based on functionality, ease of use, and target audience. Lets start with the core features of each.
Pydantic-AI
- Built on top of Pydantic, Pydantic-AI focuses on ensuring structured, validated, and type-safe outputs from LLMs.
- Its primary strength lies in converting unstructured natural language responses into well-defined Python data models using Pydantic’s validation framework.
- Ideal for applications requiring strict adherence to schemas, such as form filling, API response validation, or database population.
LangChain
- A broader framework designed to chain together multiple LLM operations into workflows.
- Supports functionalities like memory, prompt templates, and integration with external tools such as vector stores, APIs, and databases.
- Ideal for applications like chatbots, question-answering systems, and dynamic workflows that require more than validation.
Use Cases
Pydantic-AI
- Validating and parsing responses into pre-defined structures (e.g., user input forms, data pipelines).
- Applications requiring high data integrity (e.g., finance, healthcare, or legal industries).
- Developers familiar with Pydantic who want seamless integration with their Python workflows.
LangChain
- Creating conversational agents with memory and context.
- Building dynamic and multi-step workflows (e.g., summarization, retrieval-augmented generation).
- Integrating LLMs with external tools like vector databases or APIs for interactive applications.
Strengths and Weaknesses
To summarize the strengths and weaknesses of each:
Pydantic-AI
- Strengths: Simplicity, robust schema validation, seamless integration with Pydantic workflows.
- Weaknesses: Limited to validation and structuring; lacks advanced features like memory and chaining.
LangChain
- Strengths: Comprehensive framework for complex workflows, vast integrations, and modular design.
- Weaknesses: Overhead for simple tasks; requires more setup for applications focused solely on validation.
Choosing the Right Tool
While there’s some overlap between the functionalities of the two frameworks, they shine for different use cases.
When to Choose Pydantic-AI
- If your primary requirement is to validate and structure LLM outputs into predefined schemas.
- If you're already using Pydantic extensively in your projects.
- For scenarios where maintaining data integrity is critical.
When to Choose LangChain
- If you need to build complex LLM workflows involving chaining, memory, and external integrations.
- For creating applications that go beyond validation, such as chatbots or interactive systems.
- When your project involves multiple steps or integrations with external tools.
Wrapping Up
PydanticAI is a versatile framework designed to enhance AI application development through its model-agnostic and type-safe features. It supports various large language models (LLMs) like OpenAI and Gemini, allowing developers to switch between models without being tied to a specific stack. This flexibility is crucial for scalable applications. PydanticAI ensures data integrity with type-safe validation, which is essential for production-grade applications, such as customer support agents in banking.
The framework also facilitates the entire development lifecycle with features like dependency injection and Logfire integration for debugging. It enables dynamic interactions with external systems through tool-calling agents, making it suitable for applications like interactive games and customer service. PydanticAI's structured validation reduces development time and errors, offering a promising solution for reliable AI applications.
PydanticAI's capabilities make it a powerful tool for developing robust AI-driven applications, with potential for further expansion and integration with additional LLMs.
Member discussion